The header file¶
The whole G3P library implemented as a single header file that you have to include it once in your C++ code:
#include <g3p/gnuplot>
cling
include paths
cling
include pathsIf G3P is not installed in a standard folder for headers, you can add it to the cling include paths using one of the following methods:
#pragma cling add_include_path("g3p/include/path")
In a cling
REPL, but not Jupyter Notebook you can use .I
:
.I "g3p/include/path"
gnuplot
instance¶
Now you can start with a gnuplot
instance that is defined in g3p
namespace. We name our instance gp
:
g3p::gnuplot gp;
C and C++ conventions¶
There are two ways to send string literals (e.g. Gnuplot commands) and variables to a g3p
::
gnuplot
instance: C
and C++
conventions. You can even mix and match them.
Gnuplot
comes with lots of demos that you can find them here. For our first plot we use almost a verbatim copy of the Simple Plots demo. Here are the G3P versions using both conventions along with the original Gnuplot version for the sake of comparisons:
int count = 200;
gp ( "set title 'Simple Plots ( %d points )' font ',20'", count )
( "set key left box" )
( "set samples %d", count)
( "set style data points" )
( "plot [-10:10] sin(x), atan(x),cos(atan(x))" ) // <-- no semicolon
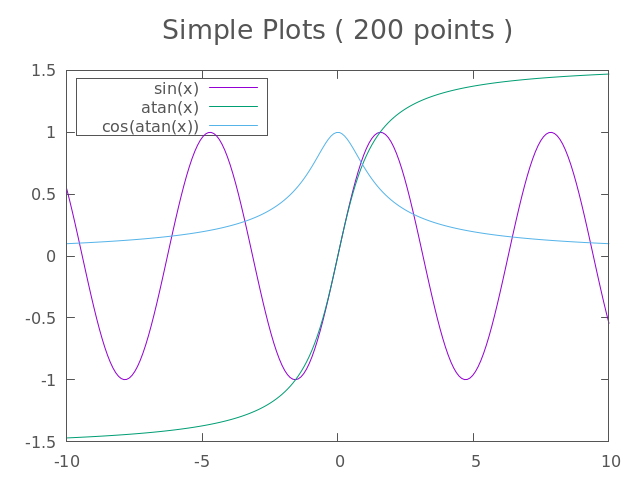
int count = 200;
gp << "set title 'Simple Plots (" << count << " points )' font ',20'\n"
<< "set key left box\n"
<< "set samples" << count << "\n"
<< "set style data points\n"
<< "plot [-10:10] sin(x), atan(x),cos(atan(x))\n" // <-- no semicolon
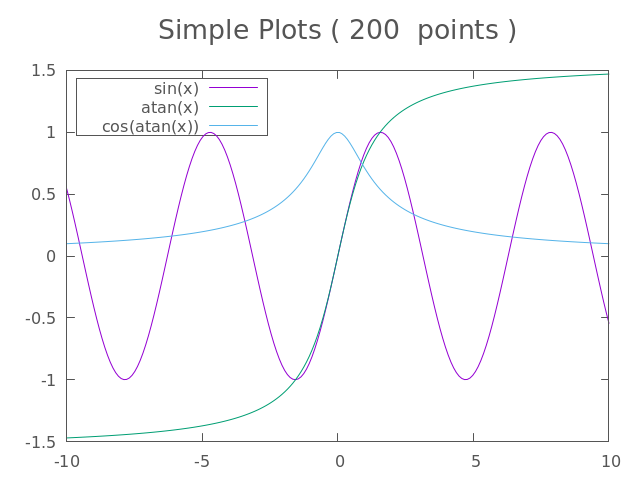
set title "Simple Plots ( 200 Points )" font ",20"
set key left box
set samples 200
set style data points
plot [-10:10] sin(x),atan(x),cos(atan(x))
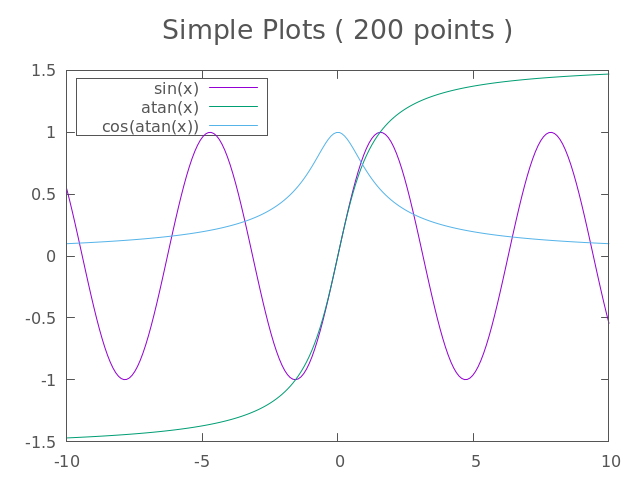
Newline¶
As you may noticed in the above examples, one important difference between C++
and C
conventions is that a newline character will be added automatically at the end of a string literal for the latter. In G3P, there are various ways to send a newline to a g3p
::
gnuplot
instance:
- Implicit
- String literal in parenthesis (i.e. overloaded function operator):
gp("...")
- String literal in parenthesis (i.e. overloaded function operator):
- Explicit
Let’s try all of the above methods in the same order for our next plot:
count = 100;
gp ( "set title 'Simple Plots ( %d points )", count ); // implicit
gp << "set key right nobox" << "\n"; // explicit - string literal
gp << "set samples" << count;
gp.endl(); // explicit - member function
gp << "plot [-pi/2:pi] cos(x),-(sin(x) > sin(x+1) ? sin(x) : sin(x+1))"
<< g3p::endl // explicit - manipulator
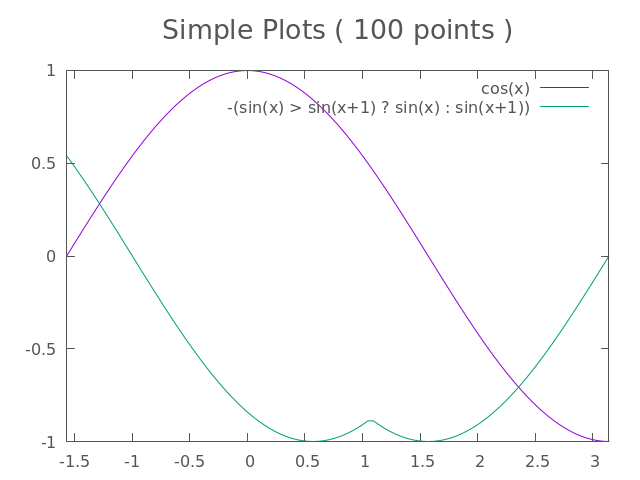
Flush¶
G3P uses buffered i/o to communicate with g3p
::
gnuplot
instances. That means the plotting commands you use in your C/C++
code are not necessarily received by the g3p
::
gnuplot
instance at any moment unless you ensure the buffer is flushed. That’s particularly important after executing plot
, splot
or replot
commands to ensure the plot is displayed. Like newline, there are various ways in G3P to flush the buffers:
- Implicit (available only in Jupyter Notebooks)
- Explicit